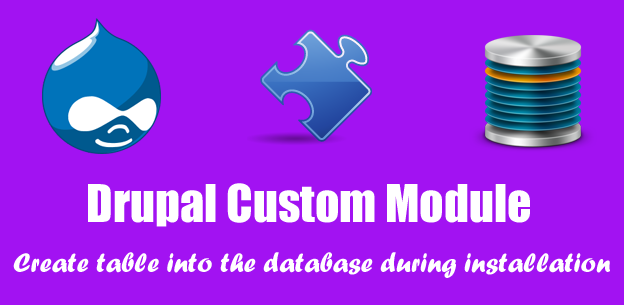
Welcome to the realm of Drupal 9 module development! In this comprehensive guide, we'll navigate the crucial steps required to craft a robust Drupal module equipped with its own database table. Buckle up as we embark on this journey, empowering you to architect modules that interact seamlessly with Drupal's database layer.
Step 1: Module Skeleton Creation
Begin by crafting the skeletal structure of your module. This includes defining the module's directory, .info.yml file, and .module file. The .info.yml file outlines essential metadata, while the .module file serves as the module's functional core.
Step 2: Define the Database Schema
Define the database schema using Drupal's Schema API. Declare the structure of your table, encompassing fields, keys, and indexes. Leverage the hook_schema() function within your .install file to articulate the database schema succinctly.
In your mymodule.install file:
use Drupal\Core\Database\Database;
/**
* Implements hook_schema().
*/
function mymodule_schema() {
$schema['mymodule_table'] = [
'description' => 'Stores data for MyModule.',
'fields' => [
'id' => [
'type' => 'serial',
'unsigned' => TRUE,
'not null' => TRUE,
'description' => 'Primary Key: Unique ID.',
],
'name' => [
'type' => 'varchar',
'length' => 255,
'not null' => TRUE,
'description' => 'Name of the entry.',
],
// Add more fields as needed.
],
'primary key' => ['id'],
];
return $schema;
}
Step 3: Module Installation Process
Implement hook_install() within your .install file to handle the installation process. This function executes when the module is installed and is ideal for executing tasks such as creating the database table defined in the schema.
In your mymodule.install file:
/**
* Implements hook_install().
*/
function mymodule_install() {
// Create the table defined in hook_schema().
// Make sure table doesnt exist in db.
\Drupal::database()->schema()->createTable('mymodule_table', mymodule_schema()['mymodule_table']);
}
Step 4: Implement Database Table Creation
Leverage Drupal's database API to create the defined database table during module installation. Utilize functions such as db_create_table() or Schema::createTable() within the hook_install() implementation to materialize the database schema.
Step 5: Uninstallation Process Management
Create an implementation of hook_uninstall() within the .install file. This function executes when the module is uninstalled and should include code to drop the module's database table using db_drop_table() or Schema::dropTable().
In your mymodule.install file:
/**
* Implements hook_uninstall().
*/
function mymodule_uninstall() {
// Drop the table when the module is uninstalled.
// Make sure table exist in db.
\Drupal::database()->schema()->dropTable('mymodule_table');
}
Step 6: Utilize the Table
With the table created and integrated into your module, harness its power within your Drupal application. Implement functionalities that interact with the database table, such as storing, retrieving, updating, or deleting data, leveraging Drupal's database abstraction layer.
Step 7: Module Testing and Validation
Validate the functionality of your module rigorously. Test various scenarios, including table creation, data manipulation, and uninstallation, ensuring robustness and adherence to Drupal coding standards.
By following these pivotal steps, you'll sculpt a formidable Drupal 9 module equipped with a purposeful database table. Empower your Drupal applications with this newfound capability, fostering dynamic interactions and seamless data management.
In your journey of Drupal module creation, the integration of a database table elevates your module's functionality, enabling it to become an integral part of the Drupal ecosystem.
Stay tuned for our upcoming articles, delving deeper into Drupal module development, where we'll explore more intricate functionalities and innovative practices.
Join us on this exhilarating path of Drupal module crafting, where your creativity merges seamlessly with Drupal's prowess, unlocking a realm of endless possibilities.
Add new comment