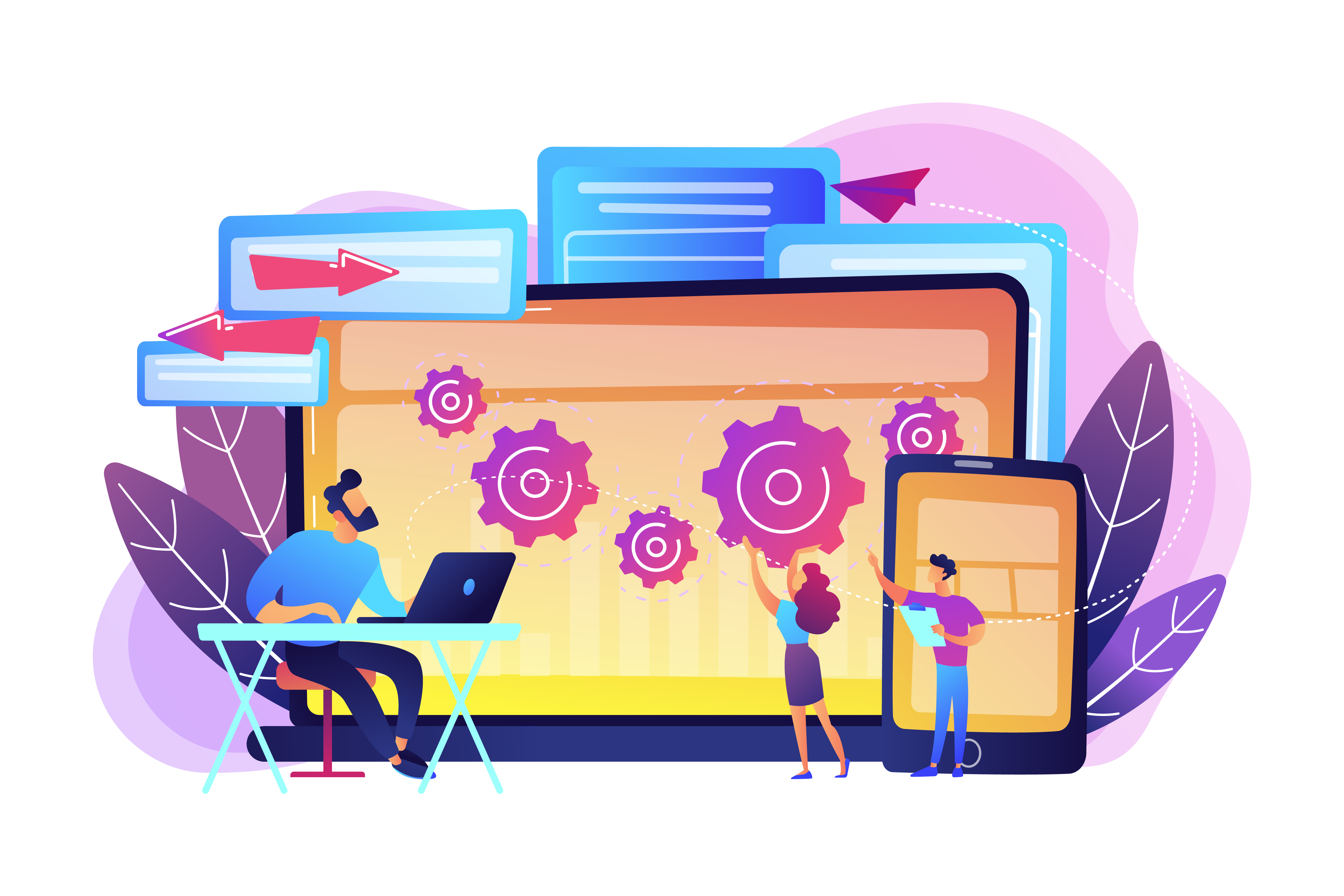
The Drupal 10 Plugin System stands as a testament to the platform's commitment to flexibility and extensibility. In this guide, we'll dive deep into the world of Drupal's Plugin System, understanding its uses, the pressing need for such a system, and the myriad benefits it offers. We'll explore scenarios where the Plugin System shines and how it empowers developers to build modular and dynamic solutions.
Unveiling the Power of the Plugin System
At the heart of Drupal's extensibility lies its Plugin System, a versatile mechanism for adding and managing functionality within modules. It offers a structured and standardized approach to extending Drupal, making it an essential tool for module developers.
Why Do We Need the Drupal 10 Plugin System?
The Growing Complexity of Drupal Modules
As Drupal websites become increasingly complex, the need for modular and maintainable code has never been more critical. Traditional approaches to module development often fall short when it comes to handling diverse functionalities. This is where the Plugin System steps in, addressing the limitations of older methods.
The Quest for Reusability
In the modern web development landscape, reusability is key. Drupal's Plugin System allows developers to create plugin types that can be reused across different modules. This reusability not only reduces redundancy but also streamlines development efforts.
Achieving a Clean and Organized Codebase
With the Plugin System, modules can neatly organize their code, keeping functionality encapsulated within plugins. This results in cleaner, more maintainable codebases that are easier to understand and extend.
Benefits of the Drupal 10 Plugin System
Modularity
Drupal's Plugin System promotes modularity by allowing developers to break down complex functionality into smaller, manageable pieces. Each piece, or plugin, serves a specific purpose, making it easier to add, remove, or swap functionality as needed.
Extensibility
The Plugin System is the cornerstone of Drupal's extensibility. Developers can create plugins without altering the core module's code, facilitating extensibility and ensuring that site customizations are preserved during updates.
Reusability
The ability to reuse plugins across different modules significantly reduces development time and effort. Developers can tap into existing plugins, accelerating the creation of new features and functionality.
Scalability
As your Drupal website evolves, the Plugin System scales with it. You can continue to add new plugins to accommodate changing requirements, ensuring your site remains flexible and adaptable.
Situations Where the Drupal Plugin System Excels
Content Types and Fields
When building modules that introduce new content types or fields, the Plugin System allows for the creation of field type plugins, making it easy to extend Drupal's content management capabilities.
Integration with External Services
Modules that interact with external APIs, payment gateways, or third-party services can benefit from the Plugin System by creating integration plugins, ensuring seamless communication.
Custom Display Formatters
Developers looking to enhance the display of content can create custom display formatter plugins, providing a rich and customizable user experience.
Tutorial: Building with the Drupal 10 Plugin System
Prerequisites
- A Drupal 10 website installed.
- Basic knowledge of PHP and Drupal module development.
Step 1: Creating a Plugin Type
In this step, we will create a new plugin type that our module will use. This defines the type of functionality we want to extend or modify within our module.
// In your Drupal module's .module file, define the plugin type.
function your_module_entity_type_build(array &$entity_types) {
$entity_types['your_plugin_type'] = [
'label' => t('Your Plugin Type'),
'handlers' => [
'list_builder' => 'Drupal\your_module\Plugin\YourPluginTypeListBuilder',
],
];
}
Explanation: In this code, we define a new plugin type called 'your_plugin_type'
. This type is given a label for clarity. We specify a list builder handler that will be responsible for managing this plugin type.
Step 2: Implementing Your Plugin
In this step, we'll implement the actual plugin. This involves creating a PHP class that extends a base plugin class and defines its behavior.
// In your Drupal module, create a folder for your plugin and define the plugin class.
namespace Drupal\your_module\Plugin\YourPluginType;
use Drupal\Core\Plugin\PluginBase;
/**
* Defines a plugin that provides custom functionality.
*
* @YourPluginType(
* id = "your_plugin_id",
* label = @Translation("Your Plugin Label"),
* )
*/
class YourPluginClassName extends PluginBase {
/**
* {@inheritdoc}
*/
public function performAction() {
// Define the behavior of your plugin here.
// This could involve processing data, rendering content, or any other custom functionality.
}
}
Explanation: In this code, we create a PHP class that extends `PluginBase`
, which is a base class for Drupal plugins. We define the plugin's ID, label, and any custom functionality it provides. The `performAction()
` method is where you would place your plugin's specific logic.
Step 3: Using Your Plugin in a Module
In this step, we'll use the plugin within a module. We'll demonstrate how to discover and invoke your plugin.
// In your Drupal module, discover and use the plugin.
use Drupal\Core\Plugin\PluginManagerBase;
use Drupal\your_module\Plugin\YourPluginType\YourPluginClassName;
// Discover and instantiate the plugin.
$plugin_manager = \Drupal::service('plugin.manager.your_plugin_type');
$plugin_instance = $plugin_manager->createInstance('your_plugin_id');
// Use the plugin's functionality.
$plugin_instance->performAction();
Explanation: In this code, we use Drupal's plugin manager to discover and instantiate the plugin we created earlier. We specify the plugin type and ID to identify the specific plugin instance. Finally, we call the `performAction()`
method to execute the plugin's custom functionality.
Lets get a better understanding with a example
Building a Shopping Cart Plugin with the Drupal 10 Plugin System
Step 1: Creating a Plugin Type
In this step, we'll define a custom plugin type for our shopping cart functionality.
// In your Drupal module's .module file, define the plugin type.
function shopping_cart_entity_type_build(array &$entity_types) {
$entity_types['shopping_cart_plugin'] = [
'label' => t('Shopping Cart Plugin'),
'handlers' => [
'list_builder' => 'Drupal\shopping_cart\Plugin\ShoppingCartListBuilder',
],
];
}
Explanation: We define a custom plugin type called 'shopping_cart_plugin'
. This type will represent the functionality of our shopping cart. We specify a list builder handler that manages this plugin type.
Step 2: Implementing the Shopping Cart Plugin
In this step, we'll implement the shopping cart plugin itself. This plugin will calculate and display the total price of items in the cart.
// In your Drupal module, create a folder for the shopping cart plugin and define the plugin class.
namespace Drupal\shopping_cart\Plugin\ShoppingCart;
use Drupal\Core\Plugin\PluginBase;
use Drupal\shopping_cart\ShoppingCartInterface;
/**
* Defines a shopping cart plugin for calculating the total price.
*
* @ShoppingCart(
* id = "shopping_cart_total_price",
* label = @Translation("Total Price Calculator"),
* )
*/
class TotalPriceCalculator extends PluginBase implements ShoppingCartInterface {
/**
* {@inheritdoc}
*/
public function calculateTotalPrice(array $items) {
$total = 0;
// Calculate the total price based on the items in the shopping cart.
foreach ($items as $item) {
$total += $item->getPrice();
}
return $total;
}
}
Explanation: We create a PHP class `TotalPriceCalculator`
that extends `PluginBase`
. This class implements the `ShoppingCartInterface`
, which defines the contract for shopping cart plugins. Our plugin calculates the total price by iterating through the cart items and summing their prices.
Step 3: Using the Shopping Cart Plugin in a Module
In this step, we'll use the shopping cart plugin within a module to calculate and display the total price.
// In your Drupal module, discover and use the shopping cart plugin.
use Drupal\Core\Plugin\PluginManagerBase;
use Drupal\shopping_cart\Plugin\ShoppingCart\TotalPriceCalculator;
// Discover and instantiate the plugin.
$plugin_manager = \Drupal::service('plugin.manager.shopping_cart_plugin');
$plugin_instance = $plugin_manager->createInstance('shopping_cart_total_price');
// Simulate cart items.
$cart_items = [
// Cart item objects with prices.
];
// Calculate the total price using the plugin.
$total_price = $plugin_instance->calculateTotalPrice($cart_items);
// Display the total price.
drupal_set_message('Total Price: ' . $total_price);
Explanation: In this code, we use Drupal's plugin manager to discover and instantiate the shopping cart plugin we created. We simulate cart items and then use the plugin to calculate the total price. Finally, we display the total price using `drupal_set_message()`
.
Conclusion
In conclusion, the Drupal 10 Plugin System is a powerful tool that empowers developers to build flexible and modular solutions. Through our example of creating a shopping cart plugin, we've seen how this system enhances extensibility, reusability, and scalability in Drupal development. With this knowledge, you're well-prepared to harness the potential of plugins and tackle complex challenges in your Drupal projects.
Add new comment