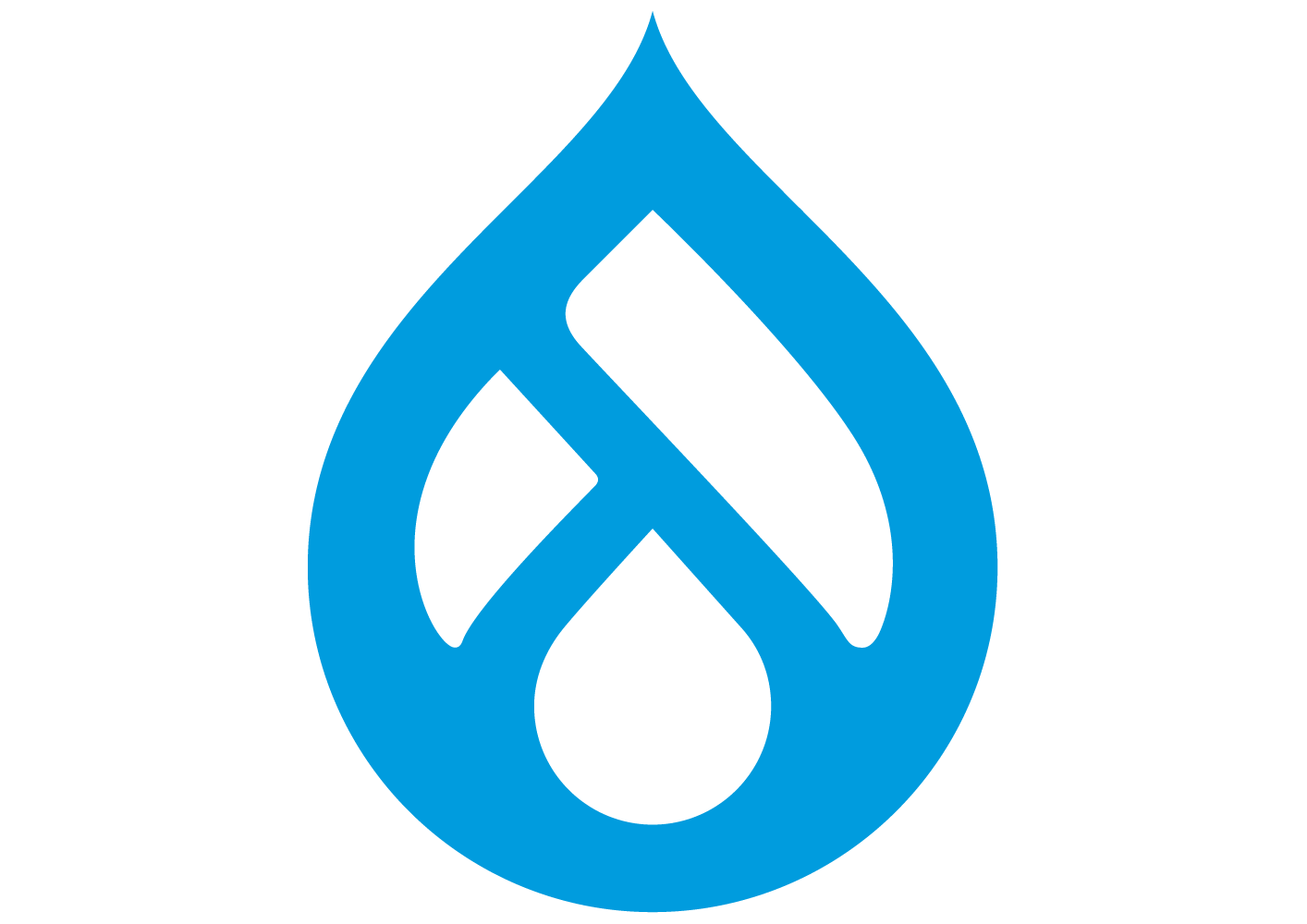
The EntityFieldManager class is a powerful tool for developers working with entity fields in Drupal. It simplifies the management of fields, their definitions, display settings, and manipulation of field instances. Understanding and utilizing this class efficiently enables developers to handle entity fields effectively within their Drupal projects.
1. Retrieving Field Definitions
The EntityFieldManager allows developers to fetch field definitions for entities, providing information about the fields attached to specific entity types.
use Drupal\Core\Entity\EntityFieldManager;
// Get the EntityFieldManager service.
$entityFieldManager = \Drupal::service('entity_field.manager');
// Get field definitions for a specific entity type.
$fieldDefinitions = $entityFieldManager->getFieldDefinitions('node', 'article');
2. Checking Field Availability
Developers can check if a specific field exists for an entity type, ensuring proper handling based on field availability.
use Drupal\Core\Entity\EntityFieldManager;
// Get the EntityFieldManager service.
$entityFieldManager = \Drupal::service('entity_field.manager');
// Check if a field exists for an entity type.
if ($entityFieldManager->hasField('node', 'field_example')) {
// Field exists, perform necessary operations.
} else {
// Field doesn't exist, handle accordingly.
}
3. Getting Display Settings
The EntityFieldManager also provides methods to retrieve display settings for fields, which can be useful for rendering fields in different contexts.
use Drupal\Core\Entity\EntityFieldManager;
// Get the EntityFieldManager service.
$entityFieldManager = \Drupal::service('entity_field.manager');
// Get display settings for a field.
$displaySettings = $entityFieldManager->getFieldDisplaySettings('node', 'article', 'field_example');
4. Manipulating Field Instances
Developers can manipulate field instances programmatically using EntityFieldManager. This includes tasks like adding, updating, or deleting field instances.
use Drupal\Core\Entity\EntityFieldManager;
// Get the EntityFieldManager service.
$entityFieldManager = \Drupal::service('entity_field.manager');
// Add a new field to an entity type.
$newField = [
'field_name' => 'field_new_example',
'entity_type' => 'node',
'type' => 'text',
// Add additional field settings as needed.
];
$entityFieldManager->addField('node', 'article', $newField);
5. Manipulating Base Fields
The EntityFieldManager also provides methods for working with base fields of entities, allowing developers to manage these fields programmatically.
use Drupal\Core\Entity\EntityFieldManager;
// Get the EntityFieldManager service.
$entityFieldManager = \Drupal::service('entity_field.manager');
// Update settings of a base field.
$entityFieldManager->updateFieldStorageDefinition('node', 'title', [
'description' => 'Custom description for the title field.',
]);
Add new comment