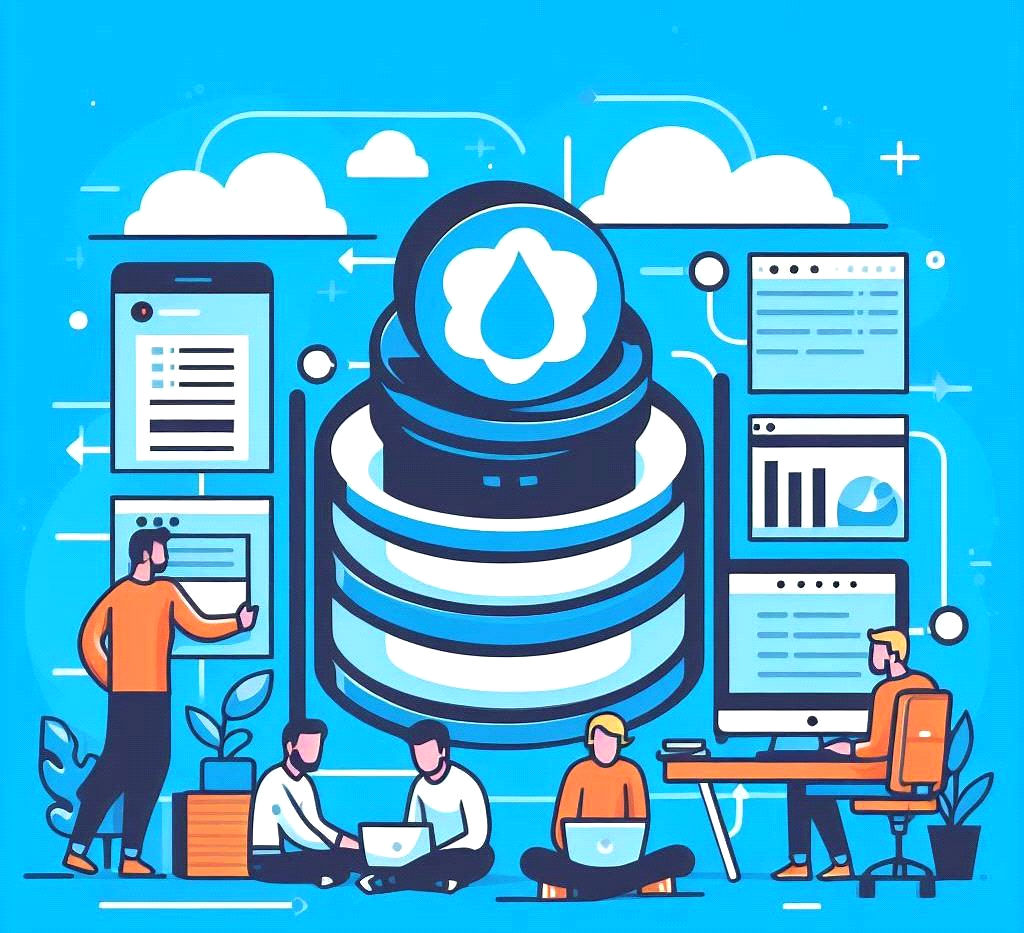
In today's web development landscape, creating and consuming RESTful APIs is crucial for building dynamic and interactive web applications. Drupal, a powerful content management system, provides robust capabilities for creating and managing APIs effortlessly. In this tutorial, we will explore the world of RESTful APIs in Drupal 9, understand their significance, and guide you through the process of creating one.
What is an API?
An API (Application Programming Interface) is a defined set of rules and protocols that allows different software applications to communicate with each other. It specifies the methods and data formats that applications can use to request and exchange information, making it possible for them to work together effectively. Think of it as a menu in a restaurant – it provides a list of dishes you can order, along with a description of each dish. When you specify which menu items you'd like, the kitchen (i.e., the system) prepares the meal and serves it to you. In this analogy, the menu is the API that defines what dishes can be ordered and how they will be prepared.
Why Use RESTful APIs in Drupal?
RESTful APIs (Representational State Transfer APIs) serve as a bridge between your Drupal site's data and external applications, allowing seamless data exchange. Here's why they are essential in Drupal development:
- Data Accessibility: APIs enable external applications to access and utilize your Drupal site's data, such as content, user information, and configuration settings.
- Integration: RESTful APIs facilitate the integration of Drupal with various platforms, such as mobile apps, third-party services, and other web applications.
- Decoupled Architectures: Drupal's API capabilities play a pivotal role in building decoupled or headless applications, where the front-end and back-end systems operate independently.
Use Cases for Drupal RESTful APIs
The flexibility of Drupal's RESTful APIs opens up numerous use cases, including:
- Mobile App Integration: Create a mobile app that pulls content from your Drupal website in real-time.
- Third-Party Integrations: Seamlessly integrate your Drupal site with external services like payment gateways or social media platforms.
- Headless Drupal: Build a decoupled front-end using technologies like React or Vue.js while relying on Drupal's backend for content management.
Now that we understand the significance and potential use cases, let's dive into the practical aspect of creating and consuming a RESTful API in Drupal 9.
Tutorial: Creating a RESTful API in Drupal
Prerequisites
Before we get started, ensure you have the following:
- A running Drupal 9 website.
- Basic knowledge of Drupal site building and content types.
- Familiarity with JavaScript for consuming the API.
Step 1: Enable the RESTful Web Services Module
- Log in to your Drupal admin dashboard.
- Navigate to
Extend
in the admin menu. - Locate and enable the
RESTful Web Services
module.
Step 2: Create a Custom Content Type
- Access
Structure
>Content types
. - Click on
Add content type
. - Create a new content type, such as "Book."
- Define fields for your content type as needed.
Step 3: Configure REST Resource
- Go to
Configuration
>Web services
>RESTful web services
. - Click
Add a resource
. - Select your custom content type (e.g., "Book").
- Configure permissions for your API resource.
Now, let's proceed to consume this API with a JavaScript application.
Example: Consuming the Drupal API
Here's a basic JavaScript example of how to consume your Drupal API:
// Define your Drupal API endpoint.
const apiUrl = 'http://your-drupal-site/api/book';
// Fetch data from the API.
fetch(apiUrl)
.then((response) => response.json())
.then((data) => {
// Handle and display the API data.
console.log(data);
})
.catch((error) => {
console.error('Error fetching data:', error);
});
This JavaScript code demonstrates the fundamental process of fetching data from a Drupal API endpoint and handling it. You can expand on this example to incorporate the retrieved data into your web application or perform more complex operations based on your project's requirements.
Here's what this code does step by step:
- Define the API Endpoint: The
apiUrl
variable is set to the URL of your Drupal API endpoint. This is where the API serves data related to books, as per the example. - Fetch Data: The
fetch
function is used to make an HTTP request to the specified API endpoint. - Process the Response: The code uses
.then
to handle the response from the API. In this case, it assumes that the API will return JSON data, so it callsresponse.json()
to parse the JSON response. - Handle Data: The data from the API is logged to the console using
console.log(data)
. You can replace this with any action you want to take with the data, such as displaying it on a web page. - Error Handling: If there is an error during the fetch request, it will be caught by the
.catch
block, and an error message will be logged to the console.
Conclusion
By following this tutorial, you've acquired the skills to create and consume RESTful APIs in Drupal 9. The ability to expose and access data via APIs enhances your Drupal website's functionality, allowing for integration with various applications and services. Whether you're building a mobile app, connecting to external platforms, or pursuing a decoupled architecture, Drupal's API capabilities empower you to achieve your development goals.
Add new comment