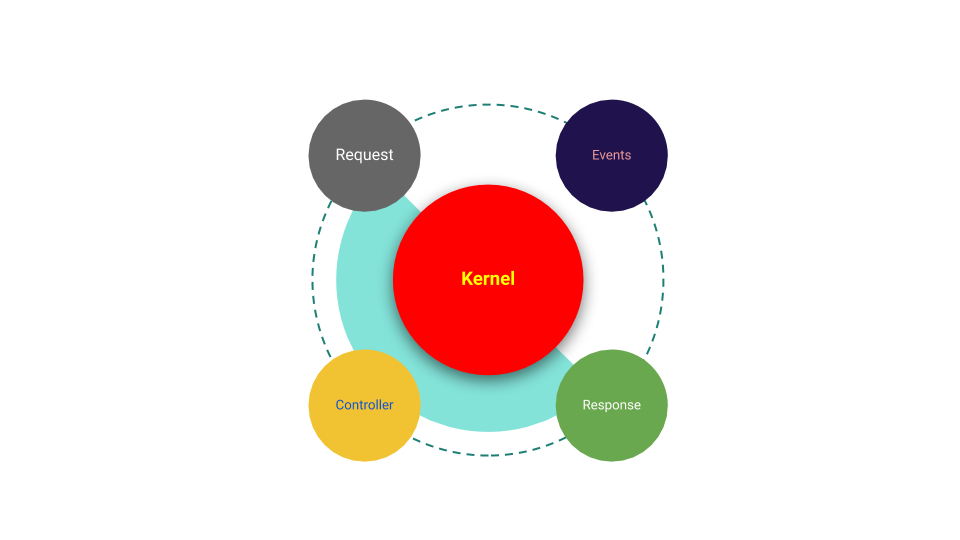
In the world of web development, handling HTTP requests is a fundamental task. Drupal 9 simplifies this process by providing the Request class, a powerful tool for processing incoming requests. In this blog post, we'll delve into the Request class in Drupal 9 and explore several ways to use it with real-world examples.
Understanding the Request Class in Drupal 9
The Request class in Drupal 9 is part of the Symfony HTTP Foundation component, offering a robust API for managing incoming HTTP requests. It provides access to various components of an HTTP request, including headers, content, query parameters, and more.
1. Accessing Request Data
One of the most common use cases for the Request class is accessing data from incoming requests. Here's an example of how to access query parameters from a URL:
use Symfony\Component\HttpFoundation\Request;
$request = Request::createFromGlobals();
$parameter = $request->query->get('param_name');
In this example, we create a Request object from the global request and then use the query attribute to access query parameters.
2. Retrieving Request Headers
You can also use the Request class to retrieve headers from incoming requests. This is useful when you need to check headers for authentication or validation. Here's an example:
use Symfony\Component\HttpFoundation\Request;
$request = Request::createFromGlobals();
$authorizationHeader = $request->headers->get('Authorization');
In this example, we create a Request object and then use the headers attribute to access the 'Authorization' header.
3. Handling POST Data
When working with forms and form submissions, you may need to access POST data from the request. Here's an example of how to do that:
use Symfony\Component\HttpFoundation\Request;
$request = Request::createFromGlobals();
$postData = $request->request->all();
In this example, we create a Request object and use the request attribute to access all POST data.
4. Checking the Request Method
The Request class allows you to check the HTTP method used in the request. This can be essential when you need to differentiate between GET and POST requests. Here's an example:
use Symfony\Component\HttpFoundation\Request;
$request = Request::createFromGlobals();
if ($request->isMethod('POST')) {
// Handle the POST request.
}
In this example, we create a Request object and use the isMethod() method to check if the request is a POST request.
5. Handling File Uploads
If you need to handle file uploads in your Drupal application, the Request class can help you manage uploaded files. Here's an example of how to access uploaded files:
use Symfony\Component\HttpFoundation\Request;
$request = Request::createFromGlobals();
$uploadedFile = $request->files->get('file_input_name');
In this example, we create a Request object and use the files attribute to access an uploaded file.
Conclusion:
The Request class in Drupal 9 is a powerful tool for managing and processing incoming HTTP requests. Whether you need to access request data, retrieve headers, handle form submissions, check request methods, or manage file uploads, the Request class provides a flexible and efficient way to work with incoming HTTP requests.
By understanding and mastering the Request class, you can build robust Drupal applications that effectively respond to various types of HTTP requests, ultimately delivering a better user experience.
Further Reading:
With these examples, you'll be well-equipped to leverage the Request class for various purposes in your Drupal 9 projects, ensuring your applications handle incoming requests with precision and efficiency.
Add new comment