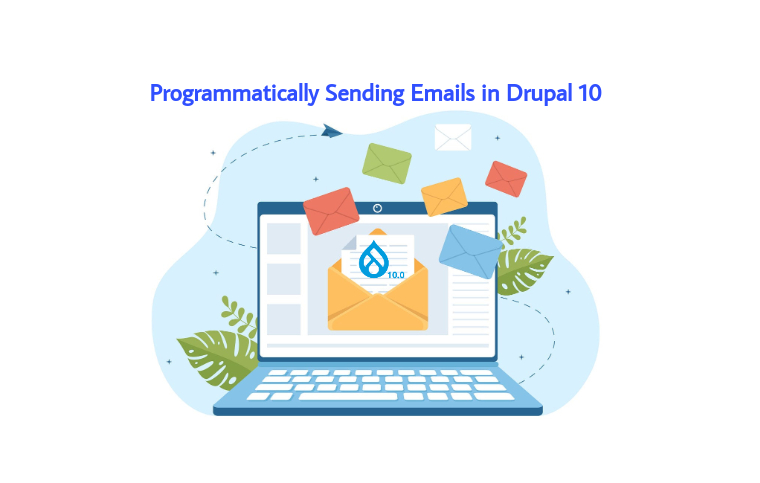
Drupal 10 provides a robust mail system that enables you to send emails programmatically within your modules or themes. This capability proves particularly useful for various purposes, such as notifying users about account creation, password resets, order confirmations, or any other event that requires email communication.
Before you begin, ensure you have the following prerequisites:
- A Drupal 10 installation
- Familiarity with PHP and Drupal development concepts
Understanding the Drupal Mail System
Drupal's mail system utilizes a plugin-based approach, allowing for flexible integration with various mail transport mechanisms, including SMTP servers and mail APIs. The core mail system provides the foundation for sending emails, while external modules can extend its functionality to support specific requirements.
Core Functions for Programmatic Email Sending
Drupal offers two primary functions for sending emails programmatically:
- drupal_mail(): This function serves as the core mechanism for sending emails. It takes an array of parameters, including the mail template identifier, recipient email address, email subject, and email body.
drupal_mail('my_module', 'welcome', 'user@example.com', language_default(), $params);
- \Drupal::service('plugin.manager.mail'): This function provides access to the mail manager service, which handles the actual sending of emails. It can be used to send emails using specific mail plugins or to perform advanced mail operations.
$mail_manager = \Drupal::service('plugin.manager.mail');
$mail_manager->mail($message, $send);
Step by step guide :-
To encapsulate the email sending logic, it's recommended to create a custom module. This allows for organized code management and easier maintenance.
- Create a new directory for your custom module, for example,
sites/all/modules/my_module
. - Inside the module directory, create a
.info.yml
file to define the module's basic information.
name: Drupal Planet Mail
type: module
description: Implements email sending functionality.
core: 10.0
- Create a
drupal_planet_mail.module
file to define the module's code.
<?php
use Drupal\Core\Mail\MailManagerInterface;
use Symfony\Component\DependencyInjection\ContainerInterface;
/**
* Implements hook_mail().
*/
function drupal_planet_mail($key, &$message, $params) {
switch ($key) {
case 'welcome':
// Define the welcome email template
$message['subject'] = t('Welcome to Our Website!');
$message['body'] = t('Hello %username,', ['%username' => $params['username']]) . PHP_EOL . PHP_EOL .
t('We hope you enjoy using our website.');
break;
}
}
Use Case :-
Sending the Welcome Email
To send the welcome email to newly created users, you can utilize the hook_user_insert() function.
function my_module_user_insert($user) {
// Get the user's email address
$email = $user->getEmail();
// Prepare the email parameters
$params = [
'username' => $user->getDisplayName(),
];
// Send the welcome email
drupal_mail('my_module', 'welcome', $email, language_default(), $params);
}
This code snippet defines a hook_user_insert()
function that is triggered whenever a new user is created. It retrieves the user's email address, prepares the email parameters, and sends the welcome email using drupal_mail()
.
Customization and Advanced Options
The Drupal mail system offers various customization options and advanced features. You can:
- Modify the email template: Create your own email templates to tailor the content and appearance of the emails.
- Add attachments: Attach files to the emails using the
$message['attachments']
property. - Use custom mail plugins: Integrate with external mail plugins for specific sending requirements.
This is how you can automate email communication within your website, enhancing user engagement and providing timely notifications. By understanding the core mail functions, utilizing hook_mail(), and exploring the available customization options, you can effectively integrate email sending capabilities into your Drupal modules and themes.
Comment
great overview, but worth…
great overview, but worth noting:
https://www.drupal.org/node/2309379
drupal_mail() is now \Drupal::service('plugin.manager.mail')->mail()
Add new comment